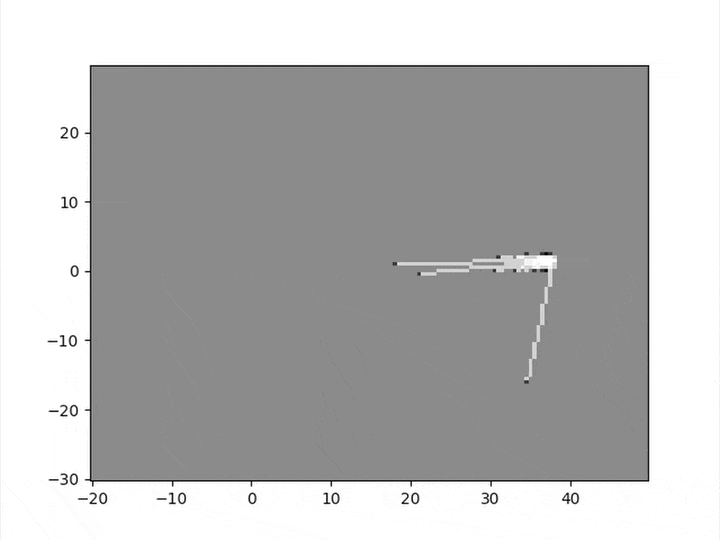
In my Robotic Localization and Mapping course, I completed a lab focused on occupancy grid mapping. The goal was to implement an algorithm that could process simulated laser range measurements and generate an accurate map of a building.
What is Occupancy Grid Mapping?
Occupancy grid mapping is a probabilistic approach used in robotics to create a map of an unknown environment. The environment is divided into a grid of cells, and each cell is assigned a probability indicating whether it is occupied (e.g., by a wall or obstacle) or free (e.g., open space). The robot uses sensor data, such as laser range measurements, to update these probabilities as it moves through the environment.
In this lab, the robot was equipped with 20 laser range finders, each pointing in a slightly different direction. As the robot moved through the building, it recorded its pose (position and orientation) and the range measurements from each laser. The challenge was to process this data and build an accurate map of the building.
Implementation Details
The occupancy grid mapping algorithm was implemented in Python, utilizing key libraries such as numpy
for matrix operations and matplotlib
for visualization. The core functionality is divided into three main classes:
OccupancyGrid
: Manages the grid structure, including cell indexing, log-odds updates, and visualization. Each cell stores the log-odds of its occupancy probability, allowing for efficient updates based on sensor measurements.RobotState
: Represents the robot’s pose (position and orientation) at any given time, which is used to process sensor data relative to the global frame.OccupancyGridMap
: Implements the mapping algorithm, including the inverse sensor model and Bresenham’s line algorithm for ray tracing. The inverse sensor model calculates log-odds updates for cells based on laser range measurements, while Bresenham’s algorithm determines which cells are intersected by each laser ray.
The algorithm processes simulated laser range data, updating the grid iteratively as the robot moves through the environment. Key parameters such as \(p_{free}\), \(p_{occup}\) , and \(p_{prior}\) were tuned to balance the confidence in free and occupied spaces, ensuring accurate map generation. The final map is visualized as a grayscale grid, with white, black, and gray cells representing free, occupied, and unknown spaces, respectively.
Results
To evaluate the impact of parameter tuning on the occupancy grid mapping algorithm, I varied the values of \(p_{free}\), \(p_{occupied}\), and \(p_{prior}\) across multiple configurations to gain insight on how the algorithm balances confidence in free and occupied spaces. Results of some of these variations can be seen below.
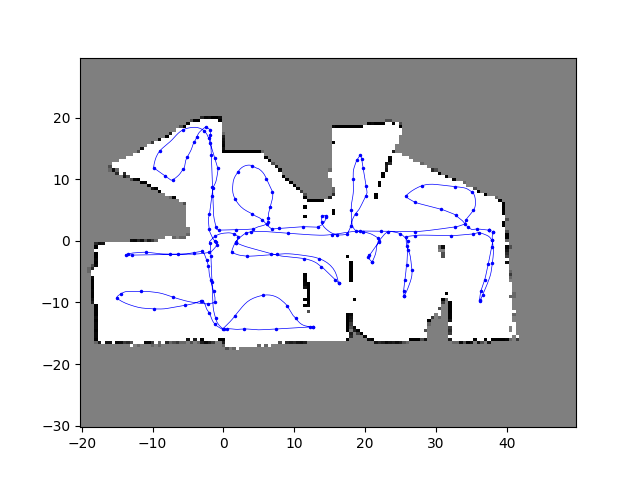
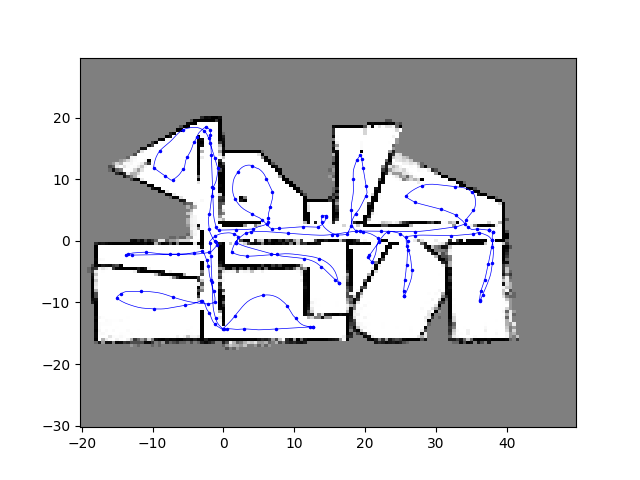
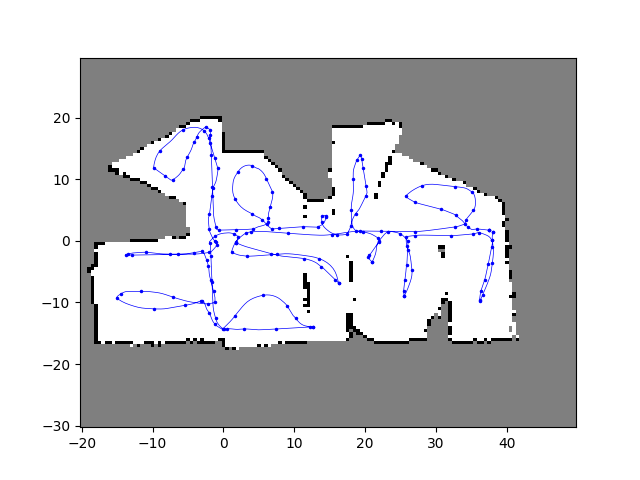
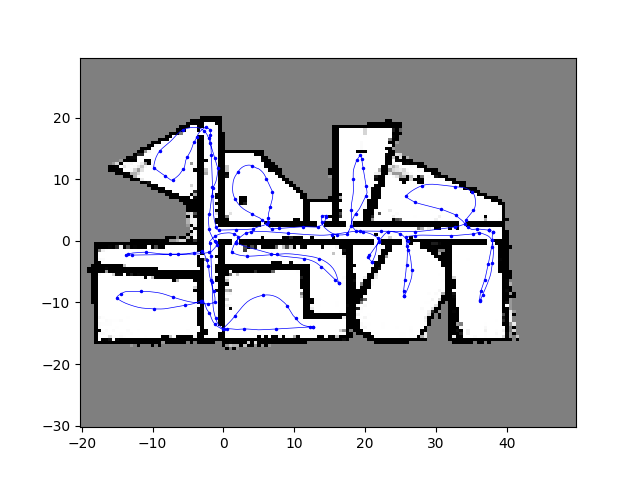